What the map method does for us can be done with a for loop. However the reason we don’t want to use a for loop is because there is room for mistakes and human made errors.
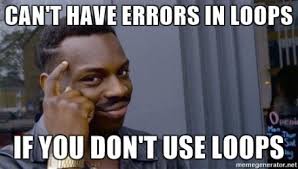
I don’t know about you but I don’t think I have written code that doesn’t use loops, well except for hello world.
Let ‘s take a look at some come that we could use the map method as opposed to a for loop.
We have an array of numbers and all we want to do is take the numbers and multiply two to each number and put the new numbers in an array.
var numbers = [1, 2, 3];
var doubledNumbers = [];
We are starting a new array because in practice we don’t want to mutate our data. We want to keep the original data and have a new array of data.
Past:
for(var i = 0; i < numbers.length; i++) {
doubledNumbers.push(numbers[i] * 2);
}
Using a for loop we can iterate through each number and push it into a new array.
Possible errors can occur if we don’t start at our zero index or if we don’t include all or if we include too many indexes to look through.
We have to use the push method to add the new numbers to the array.
Present with .map()
var doubled = numbers.map(function(number) {
return number * 2;
});
Using map all we do is pass in the function that changes the numbers and assign it to the new array. The rest is all done automatically. This is much, much easier.
Description
This will add each individual number to the new array after the math is complete.
We will always have the same amount of data in the new array as the original array.
Make sure that you use the return keywords so that the answer is pushed into the array otherwise it will add null or undefined.
Example:
We have an array of cars and each car is an object with some data.
We want to use map to iterate through each item and we want to look at just the price.
var cars = [
{model: ‘Buick’, price: ‘CHEAP’},
{model: ‘Camaro’, price: ‘expensive’}
];
var prices = cars.map(function(car) {
return car.price;
});
console.log(prices);
This is known as a pluck because we are plucking data from an object
Practical Uses:
Facebook has a feed page and you have a posts array in the back end and it has an object with an image, the title and the content.
We use a map helper to get the posts and specific information to post on the feed page.